Unleash Your Creativity with AI Code Editor: Build a Matrix Rain Effect Under an Hour!
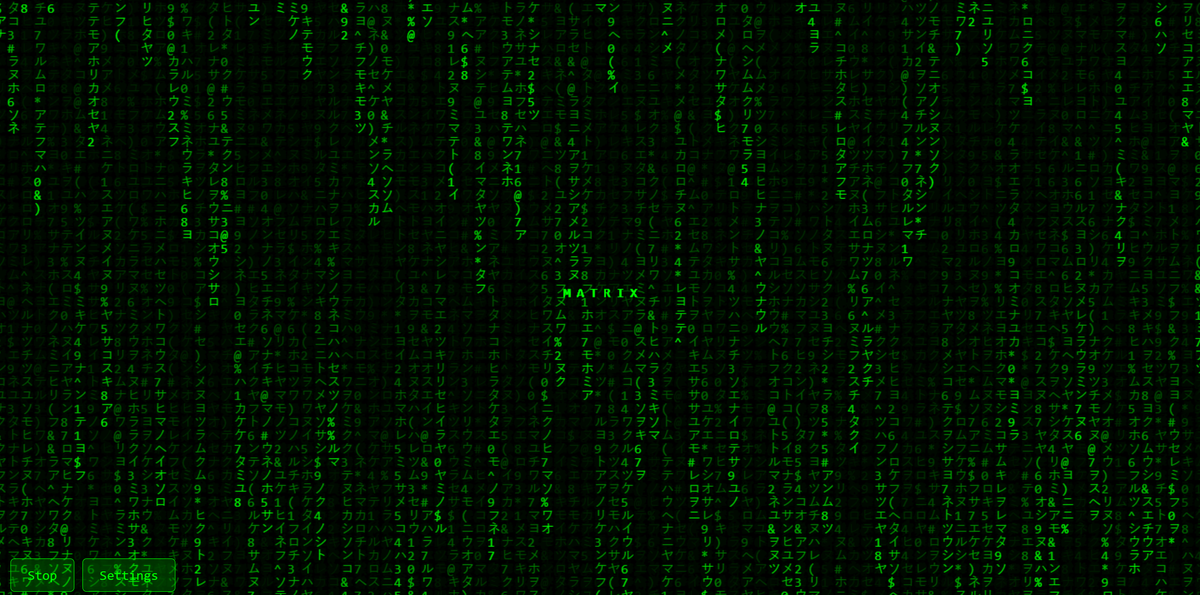
What’s stopping you from being a developer? I used to code professionally many years ago and have kept up with the latest trends, but I hadn't been actively developing for a while. Now, with tools like Cursor—an AI code editor that lets you code (or no-code) simply by asking the right questions—I can create what I want without writing a single line of code.
Out of the box, Cursor installs seamlessly. With the Pro license, I get unlimited questions and can ask continuously about exactly what I need. This setup made it incredibly easy for me to build a Matrix digital rain effect in less than one hour. Even experienced developers might spend days on a project like this, and that’s not even counting the visuals, which were generated automatically.
Live demo: https://code.feronovak.com/matrix/
The best part? You can try it even if you’re not a developer. It might be a bit harder in the beginning, but once you get the hang of asking the right questions, the creative possibilities are endless.
README.MD (generated by Cursor as well 😀)
Matrix Digital Rain Effect
A lightweight and highly customizable JavaScript library for creating the iconic Matrix digital rain effect. This implementation features configurable parameters for speed, color, text, and animations, making it easily adaptable for various use cases.
Features
- 🎨 Customizable colors with real-time updates
- ⚡ Adjustable animation speed (0.5x, 1x, 2x)
- 📝 Configurable title overlay
- 🎮 Interactive controls panel
- 🔄 Reset functionality
- 📱 Responsive design
- 🎯 Efficient canvas rendering
Implementation Overview
1. HTML Structure
The base structure uses a canvas element with data attributes for configuration:
<canvas id="matrix"
data-title="MATRIX"
data-font-size="16"
data-drop-speed="16"
data-fade-opacity="0.05"
data-fade-length="2"
data-color="#0F0"
data-frame-rate="33"
data-reset-probability="0.975"
data-characters="アイウエオカキクケコサシスセソタチツテトナニヌネノハヒフヘホマミムメモヤユヨラリルレロワヲン0123456789@#$%^&*()"
></canvas>
2. Matrix Rain Effect (script.matrix.js)
The core animation is handled by the MatrixRain
class. Key features include:
class MatrixRain {
constructor(canvas) {
this.canvas = canvas;
this.ctx = canvas.getContext('2d');
this.initialize();
}
initialize() {
// Set up canvas dimensions
this.resize();
// Initialize drops array
this.drops = [];
// Create initial drops
this.createDrops();
// Start animation loop
this.animate();
}
createDrops() {
const columns = Math.floor(this.canvas.width / this.fontSize);
for (let i = 0; i < columns; i++) {
this.drops[i] = {
x: i * this.fontSize,
y: Math.random() * -1000,
speed: this.dropSpeed,
text: this.getRandomCharacter()
};
}
}
animate() {
// Clear canvas with fade effect
this.ctx.fillStyle = `rgba(0, 0, 0, ${this.fadeOpacity})`;
this.ctx.fillRect(0, 0, this.canvas.width, this.canvas.height);
// Draw drops
this.ctx.fillStyle = this.color;
this.ctx.font = `${this.fontSize}px monospace`;
this.drops.forEach(drop => {
// Draw character
this.ctx.fillText(drop.text, drop.x, drop.y);
// Update position
drop.y += drop.speed;
// Reset drop if it's off screen
if (drop.y > this.canvas.height) {
drop.y = 0;
drop.text = this.getRandomCharacter();
}
});
// Continue animation loop
requestAnimationFrame(() => this.animate());
}
}
3. Interactive Controls (script.js)
The control panel provides user interaction:
// Default settings
const defaultSettings = {
color: '#0F0',
title: 'MATRIX',
speed: 1
};
// Speed options
const speedOptions = [
{ label: '0.5x', value: 0.5 },
{ label: '1x', value: 1 },
{ label: '2x', value: 2 }
];
// Create control elements
const controlsContainer = document.createElement('div');
controlsContainer.style.cssText = `
position: fixed;
top: 20px;
left: 20px;
display: flex;
gap: 10px;
background: rgba(0, 0, 0, 0.7);
padding: 10px;
border: 1px solid #0F0;
`;
// Add color input
const colorInput = document.createElement('input');
colorInput.type = 'text';
colorInput.value = defaultSettings.color;
colorInput.placeholder = 'Enter color (e.g., #0F0)';
// Add speed control
const speedButton = document.createElement('button');
speedButton.textContent = '1x';
speedButton.addEventListener('click', () => {
currentSpeedIndex = (currentSpeedIndex + 1) % speedOptions.length;
const option = speedOptions[currentSpeedIndex];
speedButton.textContent = option.label;
matrixRain.setSpeed(option.value * defaultSpeed);
});
4. Title Overlay
The title overlay system adds a configurable text display:
class MatrixOverlay {
constructor(canvas) {
this.canvas = canvas;
this.ctx = canvas.getContext('2d');
this.title = canvas.getAttribute('data-title') || 'MATRIX';
// Only draw title if it's not empty
if (this.title && this.title.trim()) {
this.drawTitle();
}
}
drawTitle() {
const centerX = this.canvas.width / 2;
const centerY = this.canvas.height / 2;
this.ctx.font = `${this.fontSize}px monospace`;
this.ctx.fillStyle = this.color;
this.ctx.textAlign = 'center';
this.ctx.fillText(this.title, centerX, centerY);
}
}
Styling
The Matrix theme is maintained through consistent styling:
body {
margin: 0;
overflow: hidden;
background: black;
}
button, input {
background: rgba(0, 0, 0, 0.7);
border: 1px solid #0F0;
color: #0F0;
padding: 5px 10px;
font-family: monospace;
transition: background-color 0.3s;
}
button:hover {
background: rgba(0, 255, 0, 0.2);
}
Usage
- Include the necessary files:
<script src="script.matrix.js"></script>
<script src="script.js"></script>
- Add a canvas element with desired configuration:
<canvas id="matrix" data-title="MATRIX"></canvas>
- Initialize the Matrix effect:
const canvas = document.getElementById('matrix');
const matrixRain = new MatrixRain(canvas);
Customization
The effect can be customized through data attributes or JavaScript:
data-title
: Set the overlay title textdata-color
: Change the Matrix rain colordata-drop-speed
: Adjust the falling speeddata-font-size
: Modify the character sizedata-characters
: Define custom character set
Performance Considerations
- Uses
requestAnimationFrame
for smooth animations - Implements efficient canvas clearing with fade effect
- Optimizes drop management and character updates
- Responsive to window resize events
Browser Support
Works in all modern browsers that support:
- Canvas API
- ES6 Classes
- RequestAnimationFrame